In this tutorial we will learn how to configure Qt to use OpenCV. Although the tutorial is targeted for OSX users, you can modify my suggestions for use in Linux and Windows. I assume you have a working knowledge of Qt and you have at least built a “hello world” application using it.
Of the few different ways of configuring Qt for OpenCV, we will use the one that involves pkg-config.
I have created a simple Qt application that uses OpenCV to capture frames from a webcam and display them using QGraphicsView. A screenshot is shown below.
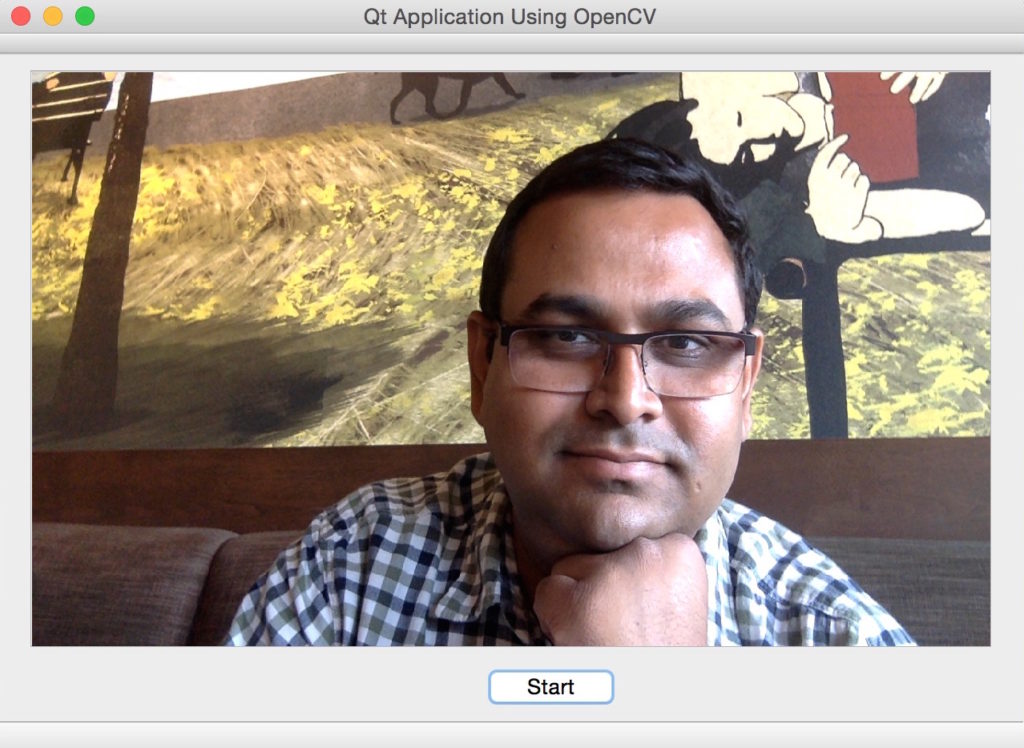
Install OpenCV
For this tutorial I am assuming you have installed OpenCV 2 or OpenCV 3. If you have not, you can install them using Homebrew. The basic installation commands are shown below.
Install OpenCV 2.4.x on OSX using Homebrew
brew tap homebrew/science
brew install opencv
Install OpenCV 3 on OSX using Homebrew
brew tap homebrew/science
brew install opencv3
You can find detailed instructions for installing OpenCV using Homebrew by clicking here. Using OpenCV 3 in a Qt application is a bit tricky for OSX because opencv and opencv3 packages contain the same libraries and so opencv3 is not deployed to /usr/local/lib like other packages. I will help you navigate through these complexities.
Install Qt Creator
You can download Qt Creator and follow the onscreen instructions for installing it. There are some restrictions on using Qt in a commercial application and you should make sure you know about licensing issues. You may find this discussion helpful.
Build settings for Qt / OpenCV project
The very first thing to do is instruct Qt where to find pkg-config. The default location for pkg-config is /usr/local/bin.
which pkg-config
# returns /usr/local/bin/pkg-config
We need to add /usr/local/bin to PATH. Go to Project, expand Build Environment and add /usr/local/bin to PATH. Don’t forget to add a colon (:) before appending /usr/local/bin! See the screenshot below.
If you are using OpenCV 3 you also need to add a new variable called PKG_CONFIG_PATH and set it to the directory that contains opencv.pc for your OpenCV 3 installation. You can find it using the following command
find /usr/local -name "opencv.pc"
# /usr/local/Cellar/opencv3/3.1.0_3/lib/pkgconfig/opencv.pc
You may not need the above step for OpenCV 2.4.x.
How to modify Qt project file ( .pro ) for OpenCV
Now that the paths are correctly set up, we need to add a few lines to our project file (.pro) to tell qmake to use pkg-config for OpenCV.
# The following lines tells Qmake to use pkg-config for opencv
QT_CONFIG -= no-pkg-config
CONFIG += link_pkgconfig
PKGCONFIG += opencv
If you are using OpenCV 3, the compiler may complain
ld: library not found for -lippicv
How to fix compiler error “ld: library not found for -lippicv”
The ippicv library the compiler is complaining about is actually included in your OpenCV installation, but it is not in the right path. Let’s first locate the library
find /usr/local/Cellar -name "libippicv*"
# /usr/local/Cellar/opencv3/3.1.0_3/share/OpenCV/3rdparty/lib/libippicv.a
You can tell the compiler where to look for libippicv.a by adding the following line to the .pro file
LIBS += -L/usr/local/Cellar/opencv3/3.1.0_3/share/OpenCV/3rdparty/lib/
Alternatively, you can symlink libippicv.a to /usr/local/lib using the following command on the terminal
ln -s /usr/local/Cellar/opencv3/3.1.0_3/share/OpenCV/3rdparty/lib/libippicv.a /usr/local/lib
and then add the following to the .pro file
LIBS += -L/usr/local/lib/
# See cautionary note below
Caution : When both opencv and opencv3 packages are installed
When both opencv and opencv3 packages are installed, and you are using opencv3 in your Qt application, adding /usr/local/lib to your path ( as shown in the previous section ) can lead to linking errors. The best thing to do in such a case is unlink opencv.
brew unlink opencv
I have spent an embarrassing amount of time debugging the above problem in El Capitan. It does not show up on Yosemite.
Runtime error on OSX El Capitan
You may receive a runtime error on El Capitan. Note the actual error message may vary sometimes.
dyld: Symbol not found: __cg_jpeg_resync_to_restart
Referenced from: /System/Library/Frameworks/ImageIO.framework/Versions/A/ImageIO
Expected in: /usr/local/lib/libJPEG.dylib
in /System/Library/Frameworks/ImageIO.framework/Versions/A/ImageIO
The program has unexpectedly finished.
To fix it go to Project -> Run -> Run Environment -> Unset DYLD_LIBRARY_PATH. See image below.
That’s all you need to know to build a Qt application with OpenCV, but let me throw in another goodie.
How to convert OpenCV Mat to QImage
The following piece of code converts OpenCV Mat to QImage. This will come in handy when you build your first Qt based OpenCV application.
# Create an OpenCV image.
Mat image(320, 240, CV_8UC3, Scalar(0,0,0));
# Convert it to QImage
QImage qImage = QImage((const unsigned char*)(image.data),
image.cols,image.rows,
QImage::Format_RGB888).rgbSwapped());