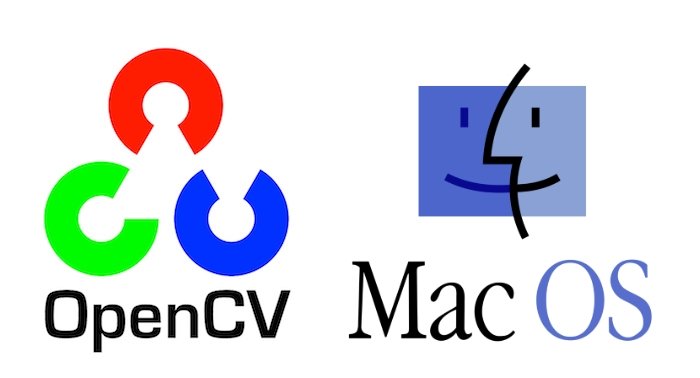
- OpenCV is now C++11 library and requires C++11-compliant compiler. Minimum required CMake version has been raised to 3.5.1.
- A lot of C API from OpenCV 1.x has been removed.
- Persistence (storing and loading structured data to/from XML, YAML or JSON) in the core module has been completely reimplemented in C++ and lost the C API as well.
- New module G-API has been added, it acts as an engine for very efficient graph-based image procesing pipelines.
- dnn module now includes experimental Vulkan backend and supports networks in ONNX format.
- The popular Kinect Fusion algorithm has been implemented and optimized for CPU and GPU (OpenCL) QR code detector and decoder have been added to the objdetect module.
- Very efficient and yet high-quality DIS dense optical flow algorithm has been moved from opencv_contrib to the video module.
1. Install XCode
Install XCode from App Store.
If XCode available on App Store is not compatible with your OS:
- Find XCode version compatible to your OS from this table https://en.wikipedia.org/w/index.php?title=Xcode#Version_comparison_table
- Go to this webpage https://developer.apple.com/download/more/
- Login if you have apple developer account else create your account and login.
- Search for xcode and download the version compatible to your OS.
- Install XCode.
- After installation open XCode, and accept xcode-build license when it asks.
2. Install OpenCV
ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)"
brew update
# Add Homebrew path in PATH
echo "# Homebrew" >> ~/.bash_profile
echo "export PATH=/usr/local/bin:$PATH" >> ~/.bash_profile
Next we will install the requirements – Python 3, CMake and Qt 5.
brew install python3
brew install cmake
brew install qt5
QT5PATH=/usr/local/Cellar/qt/5.11.2_1
We will also save current working directory in cwd variable and OpenCV version (master) in cvVersion.
cwd=$(pwd)
cvVersion="master"
# Clean build directories
rm -rf opencv/build
rm -rf opencv_contrib/build
# Create directory for installation
mkdir installation
mkdir installation/OpenCV-"$cvVersion"
Now, let’s install the Python libraries and create the Python environment.
sudo -H pip3 install -U pip numpy
# Install virtual environment
sudo -H python3 -m pip install virtualenv virtualenvwrapper
VIRTUALENVWRAPPER_PYTHON=/usr/local/bin/python3
echo "VIRTUALENVWRAPPER_PYTHON=/usr/local/bin/python3" >> ~/.bash_profile
echo "# Virtual Environment Wrapper" >> ~/.bash_profile
echo "source /usr/local/bin/virtualenvwrapper.sh" >> ~/.bash_profile
cd $cwd
source /usr/local/bin/virtualenvwrapper.sh
############ For Python 3 ############
# create virtual environment
mkvirtualenv OpenCV-"$cvVersion"-py3 -p python3
workon OpenCV-"$cvVersion"-py3
# now install python libraries within this virtual environment
pip install cmake numpy scipy matplotlib scikit-image scikit-learn ipython dlib
# quit virtual environment
deactivate
######################################
Next, let’s clone the OpenCV github repositories.
git clone https://github.com/opencv/opencv.git
cd opencv
git checkout master
cd ..
git clone https://github.com/opencv/opencv_contrib.git
cd opencv_contrib
git checkout master
cd ..
cd opencv
mkdir build
cd build
cmake -D CMAKE_BUILD_TYPE=RELEASE \
-D CMAKE_INSTALL_PREFIX=$cwd/installation/OpenCV-"$cvVersion" \
-D INSTALL_C_EXAMPLES=ON \
-D INSTALL_PYTHON_EXAMPLES=ON \
-D WITH_TBB=ON \
-D WITH_V4L=ON \
-D OPENCV_SKIP_PYTHON_LOADER=ON \
-D CMAKE_PREFIX_PATH=$QT5PATH \
-D CMAKE_MODULE_PATH="$QT5PATH"/lib/cmake \
-D OPENCV_PYTHON3_INSTALL_PATH=~/.virtualenvs/OpenCV-"$cvVersion"-py3/lib/python3.7/site-packages \
-D WITH_QT=ON \
-D WITH_OPENGL=ON \
-D OPENCV_EXTRA_MODULES_PATH=../../opencv_contrib/modules \
-D BUILD_EXAMPLES=ON ..
make -j$(sysctl -n hw.physicalcpu)
make install
cd $cwd
And that’s it! By now you should have OpenCV installed successfully in your system.
3. Test OpenCV Installation
3.1. OpenCV in Python
workon OpenCV-master-py3
Next, let’s import the module and verify the OpenCV Version installed.
import cv2
cv2.__version__
3.2. OpenCV in C++
To use OpenCV in C++, we can simply use CMakeLists.txt and specify the OpenCV_DIR variable. The format is as follows:
cmake_minimum_required(VERSION 3.1)
# Enable C++11
set(CMAKE_CXX_STANDARD 11)
set(CMAKE_CXX_STANDARD_REQUIRED TRUE)
SET(OpenCV_DIR /installation/OpenCV-master/lib/cmake/opencv4)
Make sure that you replace OpenCV_Home_Dir with correct path. For example, in my case:
SET(OpenCV_DIR /usr/local/Cellar/OpenCV_installation/installation/OpenCV-master/lib/cmake/opencv4)
Once you have made your CMakeLists.txt, follow the steps given below.
mkdir build && cd build
cmake ..
cmake --build . --config Release
This will generate your executable file in build directory.
Hope this script proves to be useful for you :). Stay tuned for more interesting stuff. In case of any queries, feel free to comment below and we will get back to you as soon as possible.